Alternatively, updates to records can be handles using Ribbon Buttons, and Client Site JavaScript. This approach can also provide additional flexibility in allowing records to be processed as a group, instead of individually.
This following example shows the process of updating a field on Work Order Products that are shown on a Sub-Grid on a Work Order form.
Creating a JavaScript Library and Function
The following temporary function is created to test if record details are being passed successfully and we can see the exact structure of the products data array.
function updateWOPs(products) {
console.log(products);
}
Create a Ribbon Button
First, create a temporary solution, and add the Table for the records that will be selected.
When adding, make sure that no other complaints are added
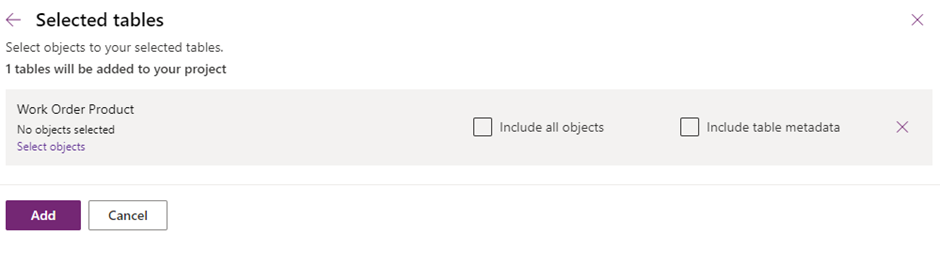
In the Solutions Elements section, create a new Command.

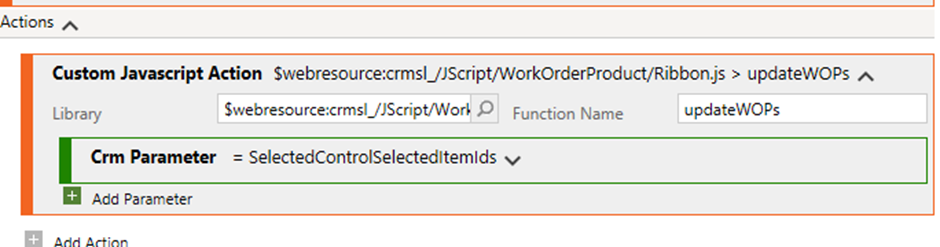
In the Enable Rules section, press the Add Enable Rule button, and select a New Rule. Press the Add Step button and choose Selected Count Rule. Enter 1 for the Minimum Field. This will cause the Ribbon button to only show if there are one or more records selected.
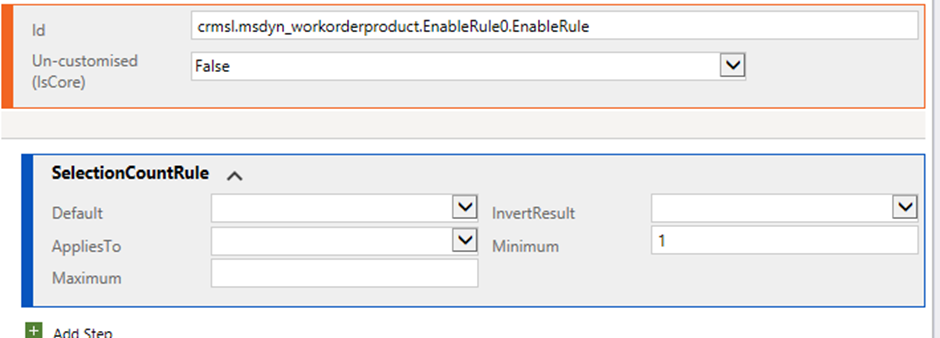
Drag a “Button” form the Toolbox and place it to the required button group.
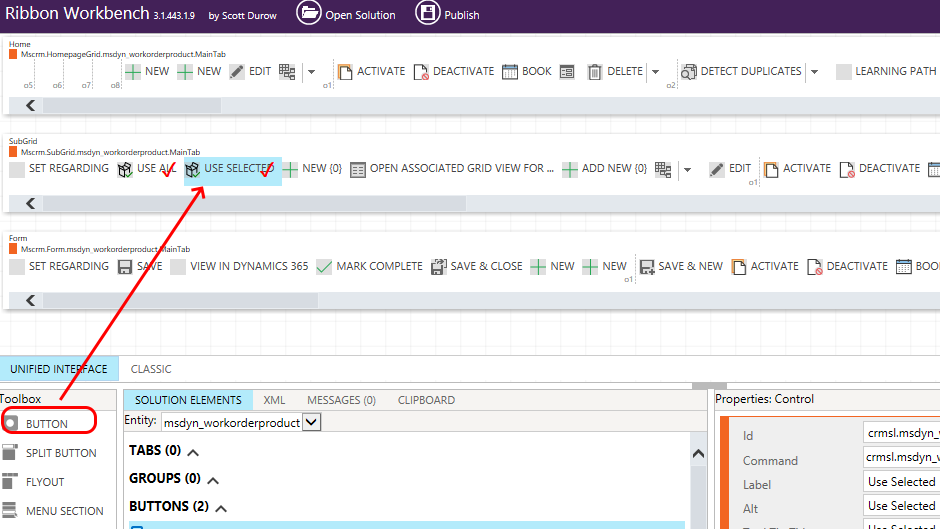
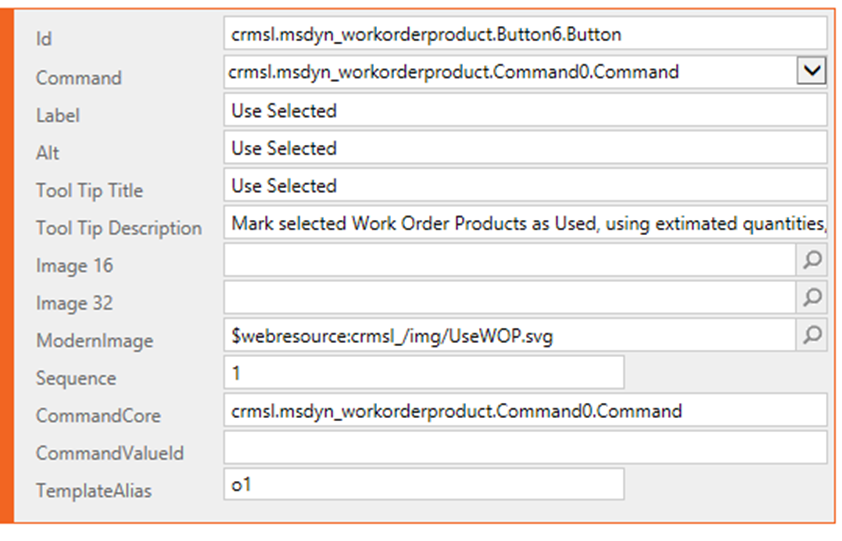
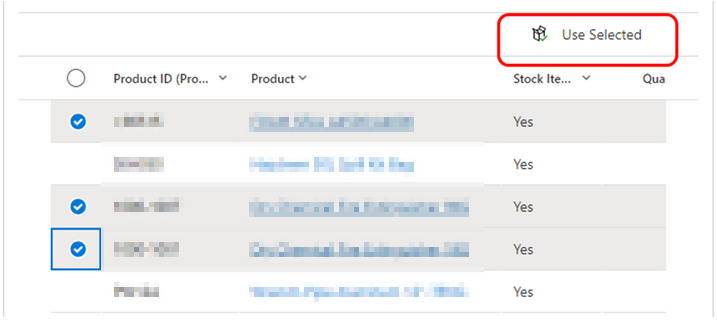
Processing Results
For this example, we will make a simple update to each record through the Web API interface. Here is the updated JavaScript function:
function updateWOPs(products) {
‘use strict’;
if (products.length > 0) {
var subgrid = Xrm.Page.ui.controls.get(“workorderproductsgrid”);
var record = {};
record.msdyn_linestatus = 690970001; // Used
products.forEach((product) => {
Xrm.WebApi.online.updateRecord(“msdyn_workorderproduct”, product, record).then(
function success(result) {
},
function (error) {
Xrm.Navigation.openAlertDialog(error.message);
}
);
});
subgrid.refresh();
}
}
Extra Notes
- Records that are passed from a list of sub-grids are limited to the records that are displayed on the form, and don’t include any records on other pages. This is true even when clicking ‘selects all’ for the list. So, for example, If there are 15 records, but a sub-grid is set to only show 10 records on two pages, then a maximum of 10 records can be selected and passed to the JavaScript function.
- The are several other parameters that can be passed to the JavaScript function, such as “SelectedControlAllItemIds” and “SelectedControlAllItemCount”, but these will also be limited to the records shown on the current page. To get a true count of all the related records, they will need to be retrieved from the JavaScript function.